簡介
消息驅動是一種進程/線程的運行模式,內部或者外部的消息事件被放到進程/線程的消息隊列中按序處理是現在的操作系統普遍采用的機制.Android也是采用了消息驅動的機制來處理各種外部按鍵,觸屏,系統Intent,廣播事件等消息.
Android的消息隊列是線程相關的,每啟動一個線程,都可以在內部創建一個消息隊列,然后在消息隊列中不斷循環檢查是否有新的消息需要處理,如果有,則對該消息進行處理,如果沒有,線程就進入休眠狀態直到有新的消息需要處理為止.
數據模型
Android中與消息機制相關的類主要有Looper,MessageQueue,Handler,Message,相關的代碼主要在以下文件中:
- frameworks/base/core/java/android/os/Looper.java
- frameworks/base/core/java/android/os/Message.java
- frameworks/base/core/java/android/os/MessageQueue.java
- frameworks/base/core/java/android/os/Handler.java
- frameworks/base/core/jni/android_os_MessageQueue.cpp
- system/core/libutils/Looper.cpp
- Looper
Looper對象是用來創建消息隊列并進入消息循環處理的.每個線程只能有一個Looper對象,同時對應著一個MessageQueue,發送到該線程的消息都將存放在該隊列中,并由Looper循環處理。Android默認只為主線程)(UI線程)創建了Looper,所以當我們新建線程需要使用消息隊列時必須手動創建Looper. - MessageQueue
MessageQueue即消息隊列,由Looper創建管理,一個Looper對象對應一個MessageQueue對象. - Handler
Handler是消息的接收與處理者,Handler將Message添加到消息隊列,同時也通過Handler的回調方法handleMessage()處理對應的消息.一個Handler對象只能關聯一個Looper對象,但多個Handler對象可以關聯到同一個Looper.默認情況下Handler會關聯到實例化Handler線程的Lopper,也可以通過Handler的構造函數的Looper參數指定Handler關聯到某個線程的Looper,即發送消息到某個指定線程并在該線程中回調Handler處理該消息. - Message
Message是消息的載體,Parcelable的派生類,通過其成員變量target關聯到Handler對象.
它們之間關系如下圖示:
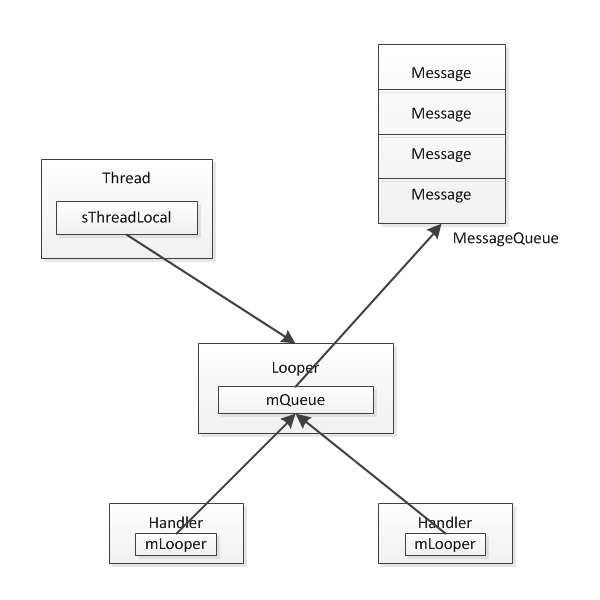
在代碼中我們一般如下使用線程的消息機制:
class LooperThread extends Thread {
public Handler mHandler;
public void run() {
Looper.prepare();
mHandler = new Handler() {
public void handleMessage(Message msg) {
// process incoming messages here
}
};
Looper.loop();
}
}
線程消息隊列的創建
線程的消息隊列通過Looper創建并維護的,主線程中調用Looper.prepareMainLooper(),其他子線程中調用Looper.prepare()來創建消息隊列.一個線程多次調用prepareMainLooper()或prepare()將會拋出異常.
在介紹消息隊列創建之前,首先了解一下Looper與MessageQueue,再看消息隊列創建的流程.
- Looper類的主要成員變量與方法如下:
public final class Looper {
static final ThreadLocal<Looper> sThreadLocal = new ThreadLocal<Looper>();
private static Looper sMainLooper;
final MessageQueue mQueue;
final Thread mThread;
public static void prepare() {...}
private static void prepare(boolean quitAllowed) {...}
public static void prepareMainLooper() {...}
public static Looper getMainLooper() {...}
public static void loop() {...}
}
- sThreadLocal是靜態成員變量,用于保存線程私有的Looper對象
- sMainLooper是主線程的Looper對象.在prepareMainLooper()中賦值,可通過調用getMainLooper獲取
- mQueue即消息隊列,在Looper構造函數中初始化
- mThread即Looper所在的線程
- MessageQueue類的主要成員變量與方法如下:
public final class MessageQueue {
private final boolean mQuitAllowed;
private long mPtr;
Message mMessages;
MessageQueue(boolean quitAllowed) {...}
boolean enqueueMessage(Message msg, long when) {...}
Message next() {...}
}
- mQuitAllowed代表是否允許退出消息循環,主線程中默認為false,子線程默認false
- mPtr保存的是NativeMessageQueue的地址,通過該地址就可以找到java層MessageQueue所對應native的MessageQueue.
- mMessages即消息隊列,通過mMessages可以遍歷整個消息隊列
- 消息隊列的創建:
消息隊列的創建從Looper.prepare()/Looper.prepareMainLooper()開始
public static void prepare() {
prepare(true);
}
public static void prepareMainLooper() {
prepare(false);
synchronized (Looper.class) {
if (sMainLooper != null) {
throw new IllegalStateException("The main Looper has already been prepared.");
}
sMainLooper = myLooper();
}
}
private static void prepare(boolean quitAllowed) {
if (sThreadLocal.get() != null) {
throw new RuntimeException("Only one Looper may be created per thread");
}
sThreadLocal.set(new Looper(quitAllowed));
}
private Looper(boolean quitAllowed) {
mQueue = new MessageQueue(quitAllowed);
mThread = Thread.currentThread();
}
通過調用prepare()或prepareMainLooper()創建Looper對象,然后保存到sThreadLocal中,sThreadLocal是模板類ThreadLocal<T>,它通過線程ID與對象關聯的方式實現線程本地存儲功能.這樣放入sThreadLocal對象中的Looper對象就與創建它的線程關聯起來了.所以可以從sThreadLocal中獲取到保存的Looper對象:
public static @Nullable Looper myLooper() {
return sThreadLocal.get();
}
主線程的Loopper對象保存在sMainLooper,可以通過getMainLooper獲取
public static Looper getMainLooper() {
synchronized (Looper.class) {
return sMainLooper;
}
}
創建Looper同時會創建Looper關聯的MessageQueue并賦值給成員變量mQueue,接下來再看new MessageQueue(quitAllowed)的過程:
MessageQueue(boolean quitAllowed) {
mQuitAllowed = quitAllowed;
mPtr = nativeInit();
}
可以看到,直接調用了nativeInit().這個JNI方法定義在android_os_MessageQueue.cpp
static jlong android_os_MessageQueue_nativeInit(JNIEnv* env, jclass clazz) {
NativeMessageQueue* nativeMessageQueue = new NativeMessageQueue();
if (!nativeMessageQueue) {
jniThrowRuntimeException(env, "Unable to allocate native queue");
return 0;
}
nativeMessageQueue->incStrong(env);
return reinterpret_cast<jlong>(nativeMessageQueue);
}
nativeInit()中首先創建了nativeMessageQueue,然后又將nativeMessageQueue的地址賦值給java層的mPtr,所以java層的MessageQueue就可以通過mPtr找到nativeMessageQueue了.
再看new NativeMessageQueue()過程,NativeMessageQueue的構造如下:
NativeMessageQueue::NativeMessageQueue() : mInCallback(false), mExceptionObj(NULL) {
mLooper = Looper::getForThread();
if (mLooper == NULL) {
mLooper = new Looper(false);
Looper::setForThread(mLooper);
}
}
它首先通過Looper::getForThread()判斷當前線程是否已創建過Looper對象,如果沒有則創建.注意,這個Looper對象是實現在JNI層的,與上面Java層的Looper是不一樣的,不過也是對應的關系.JNI層的Looper對象的創建過程是在Looper.cpp中實現的.
Looper::Looper(bool allowNonCallbacks) :
mAllowNonCallbacks(allowNonCallbacks), mSendingMessage(false),
mPolling(false), mEpollFd(-1), mEpollRebuildRequired(false),
mNextRequestSeq(0), mResponseIndex(0), mNextMessageUptime(LLONG_MAX) {
mWakeEventFd = eventfd(0, EFD_NONBLOCK);
LOG_ALWAYS_FATAL_IF(mWakeEventFd < 0, "Could not make wake event fd. errno=%d", errno);
AutoMutex _l(mLock);
rebuildEpollLocked();
}
創建eventfd并賦值給mWakeEventFd,在以前的Android版本上,這里創建的是pipe管道.eventfd是較新的API,被用作一個事件等待/響應,實現了線程之間事件通知.
void Looper::rebuildEpollLocked() {
// Close old epoll instance if we have one.
if (mEpollFd >= 0) {
#if DEBUG_CALLBACKS
ALOGD("%p ~ rebuildEpollLocked - rebuilding epoll set", this);
#endif
close(mEpollFd);
}
// Allocate the new epoll instance and register the wake pipe.
mEpollFd = epoll_create(EPOLL_SIZE_HINT);
LOG_ALWAYS_FATAL_IF(mEpollFd < 0, "Could not create epoll instance. errno=%d", errno);
struct epoll_event eventItem;
memset(& eventItem, 0, sizeof(epoll_event)); // zero out unused members of data field union
eventItem.events = EPOLLIN;
eventItem.data.fd = mWakeEventFd;
int result = epoll_ctl(mEpollFd, EPOLL_CTL_ADD, mWakeEventFd, & eventItem);
LOG_ALWAYS_FATAL_IF(result != 0, "Could not add wake event fd to epoll instance. errno=%d",
errno);
for (size_t i = 0; i < mRequests.size(); i++) {
const Request& request = mRequests.valueAt(i);
struct epoll_event eventItem;
request.initEventItem(&eventItem);
int epollResult = epoll_ctl(mEpollFd, EPOLL_CTL_ADD, request.fd, & eventItem);
if (epollResult < 0) {
ALOGE("Error adding epoll events for fd %d while rebuilding epoll set, errno=%d",
request.fd, errno);
}
}
}
rebuildEpollLocked中通過epoll_create創建了一個epoll專用的文件描述符,EPOLL_SIZE_HINT表示mEpollFd上能監控的最大文件描述符數.最后調用epoll_ctl監控mWakeEventFd文件描述符的EPOLLIN事件,即當eventfd中有內容可讀時,就喚醒當前正在等待的線程.
C++層的這個Looper對象創建好了之后,就返回到JNI層的NativeMessageQueue的構造函數,再返回到Java層的消息隊列MessageQueue的創建過程,最后從Looper的構造函數中返回.線程消息隊列的創建過程也就此完成.
總結一下:
- 首先在Java層創建了一個Looper對象,然后創建MessageQueue對象mQueue,進入MessageQueue的創建過程
- MessageQueue在JNI層創建了一個NativeMessageQueue對象,并將這個對象保存在MessageQueue的成員變量mPtr中
- 在JNI層,創建了NativeMessageQueue對象時,會創建了一個Looper對象,保存在JNI層的NativeMessageQueue對象的成員變量mLooper中,這個對象的作用是,當Java層的消息隊列中沒有消息時,就使Android應用程序線程進入等待狀態,而當Java層的消息隊列中來了新的消息后,就喚醒Android應用程序的線程來處理這個消息
-
關于java層與JNI層的Looper,MessageQueue對象可以這樣理解,java層的Looper,MessageQueue主要實現了消息隊列發送處理邏輯,而JNI層的主要實現是線程的等待/喚醒.在邏輯上他們還是一一對應的關系,只不過側重點不同.
java與jni層Looper,MessageQueue關系
線程消息隊列的循環
當線程消息隊列創建完成后,即進入消息隊列循環處理過程中,Android消息隊列的循環通過Loop.Loop()來實現,整個流程如下圖示.
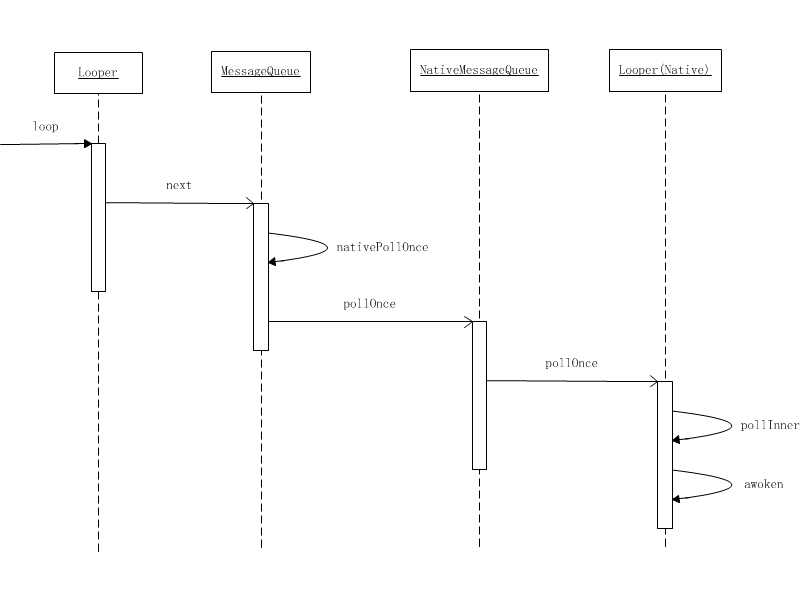
下面具體來看具體分析
public static void loop() {
final Looper me = myLooper();
if (me == null) {
throw new RuntimeException("No Looper; Looper.prepare() wasn't called on this thread.");
}
final MessageQueue queue = me.mQueue;
...
for (;;) {
Message msg = queue.next(); // might block
if (msg == null) {
// No message indicates that the message queue is quitting.
return;
}
...
msg.target.dispatchMessage(msg);
...
}
}
進入loop前,首先通過myLooper()拿到前面創建的Looper對象,如果為null將會拋出異常,這也就是為什么必須在Looper.loop()之前調用Looper.prepare()或者Looper.prepareMainLooper()的原因.接下來通過me.mQueue拿到MessageQueue對象,而后進入到無盡循環處理中.在循環中通過queue.next()從隊列中取消息,再調用msg.target.dispatchMessage(msg)處理.下面看一下queue.next()流程.
Message next() {
final long ptr = mPtr;
if (ptr == 0) {
return null;
}
int pendingIdleHandlerCount = -1;
int nextPollTimeoutMillis = 0;
for (;;) {
if (nextPollTimeoutMillis != 0) {
Binder.flushPendingCommands();
}
nativePollOnce(ptr, nextPollTimeoutMillis);
synchronized (this) {
final long now = SystemClock.uptimeMillis();
Message prevMsg = null;
Message msg = mMessages;
if (msg != null && msg.target == null) {
do {
prevMsg = msg;
msg = msg.next;
} while (msg != null && !msg.isAsynchronous());
}
if (msg != null) {
if (now < msg.when) {
nextPollTimeoutMillis = (int) Math.min(msg.when - now, Integer.MAX_VALUE);
} else {
mBlocked = false;
if (prevMsg != null) {
prevMsg.next = msg.next;
} else {
mMessages = msg.next;
}
msg.next = null;
if (false) Log.v("MessageQueue", "Returning message: " + msg);
return msg;
}
} else {
nextPollTimeoutMillis = -1;
}
if (mQuitting) {
dispose();
return null;
}
if (pendingIdleHandlerCount < 0
&& (mMessages == null || now < mMessages.when)) {
pendingIdleHandlerCount = mIdleHandlers.size();
}
if (pendingIdleHandlerCount <= 0) {
mBlocked = true;
continue;
}
if (mPendingIdleHandlers == null) {
mPendingIdleHandlers = new IdleHandler[Math.max(pendingIdleHandlerCount, 4)];
}
mPendingIdleHandlers = mIdleHandlers.toArray(mPendingIdleHandlers);
}
for (int i = 0; i < pendingIdleHandlerCount; i++) {
final IdleHandler idler = mPendingIdleHandlers[i];
mPendingIdleHandlers[i] = null;
boolean keep = false;
try {
keep = idler.queueIdle();
} catch (Throwable t) {
Log.wtf("MessageQueue", "IdleHandler threw exception", t);
}
if (!keep) {
synchronized (this) {
mIdleHandlers.remove(idler);
}
}
}
pendingIdleHandlerCount = 0;
nextPollTimeoutMillis = 0;
}
}
先看一下開始定義的2個變量的含義,pendingIdleHandlerCount表示消息隊列空閑消息處理器(IdleHandler)的個數,nextPollTimeoutMillis表示沒有消息處理時,線程需睡眠等待的時間.nativePollOnce將會睡眠等待nextPollTimeoutMillis時間.從nativePollOnce返回后,再從消息隊列中取消息,如果沒有任何消息,那么nextPollTimeoutMillis賦值為-1,表示下一次nativePollOnce無限制等待直到其他線程把它喚醒.如果取到消息,比較消息處理的時間與當前時間,如果消息處理的時間未到(now < msg.when),那么計算nextPollTimeoutMillis,等下一次時間到時再處理.如果消息處理時間已到,那么取出消息返回到Looperde的loop中處理.另外如果當前沒有消息處理時,會回調注冊的IdleHandler.
下面繼續分析nativePollOnce.
static void android_os_MessageQueue_nativePollOnce(JNIEnv* env, jobject obj,
jlong ptr, jint timeoutMillis) {
NativeMessageQueue* nativeMessageQueue = reinterpret_cast<NativeMessageQueue*>(ptr);
nativeMessageQueue->pollOnce(env, obj, timeoutMillis);
}
void NativeMessageQueue::pollOnce(JNIEnv* env, jobject pollObj, int timeoutMillis) {
mPollEnv = env;
mPollObj = pollObj;
mLooper->pollOnce(timeoutMillis);
mPollObj = NULL;
mPollEnv = NULL;
if (mExceptionObj) {
env->Throw(mExceptionObj);
env->DeleteLocalRef(mExceptionObj);
mExceptionObj = NULL;
}
}
最終nativePollOnce調用的JNI層Looper的pollOnce
int Looper::pollOnce(int timeoutMillis, int* outFd, int* outEvents, void** outData) {
int result = 0;
for (;;) {
...
if (result != 0) {
...
return result;
}
result = pollInner(timeoutMillis);
}
}
在pollOnce中不斷的循環調用pollInner來檢查線程是否有新消息需要處理.如果有新消息處理或者timeoutMillis時間到,則返回到java層MessageQueue的next()繼續執行.
int Looper::pollInner(int timeoutMillis) {
...
int result = POLL_WAKE;
struct epoll_event eventItems[EPOLL_MAX_EVENTS];
int eventCount = epoll_wait(mEpollFd, eventItems, EPOLL_MAX_EVENTS, timeoutMillis);
...
for (int i = 0; i < eventCount; i++) {
int fd = eventItems[i].data.fd;
uint32_t epollEvents = eventItems[i].events;
if (fd == mWakeEventFd) {
if (epollEvents & EPOLLIN) {
awoken();
} else {
ALOGW("Ignoring unexpected epoll events 0x%x on wake event fd.", epollEvents);
}
} else {
...
}
}
...
return result;
}
epoll_wait會監聽前面創建的epoll實例的文件描述符上的IO讀寫事件,如果文件描述上沒有IO事件出現,那么則等待timeoutMillis延時,檢測到EPOLLIN事件即文件描述符上發生了寫事件,隨后調用awoken讀出數據,以便接收新的數據.
void Looper::awoken() {
uint64_t counter;
TEMP_FAILURE_RETRY(read(mWakeEventFd, &counter, sizeof(uint64_t)));
}
在awoken中讀出數據.然后一步步返回到java層的MessageQueue繼續消息處理.
線程消息的發送
消息的發送是通過Handler來執行的,下面我們從new Handler()開始,一步步分析消息的發送過程
首先看一下Handler類的主要數據成員與方法:
public class Handler {
final MessageQueue mQueue;
final Looper mLooper;
public Handler() {...}
public Handler(Looper looper, Callback callback) {...}
private boolean enqueueMessage(MessageQueue queue, Message msg, long uptimeMillis) {...}
public void handleMessage(Message msg) {...}
public final boolean sendMessage(Message msg){...}
public final boolean sendEmptyMessage(int what){...}
public final boolean sendEmptyMessageAtTime(int what, long uptimeMillis) {...}
public final boolean sendEmptyMessageDelayed(int what, long delayMillis) {...}
public boolean sendMessageAtTime(Message msg, long uptimeMillis) {...}
...
public final boolean post(Runnable r){...}
public final boolean postAtFrontOfQueue(Runnable r){...}
public final boolean postAtTime(Runnable r, long uptimeMillis){...}
public final boolean postDelayed(Runnable r, long delayMillis){...}
}
- mQueue handler對應的MessageQueue對象,通過handler發送的消息都將插入到mQueue隊列中
- mLooper handler對應的Looper對象,如果創建Handler前沒有實例化Looper對象將拋出異常.
Handler是與Looper對象相關聯的,我們創建的Handler對象都會關聯到某一Looper,默認情況下,Handler會關聯到創建Handler對象所在線程的Looper對象,也可通過Handler的構造函數來指定關聯到的Looper.Handler發送消息有二類接口,post類與send類,一般send類用來發送傳統帶消息ID的消息,post類用來發送帶消息處理方法的消息.
下面來看消息發送的具體流程
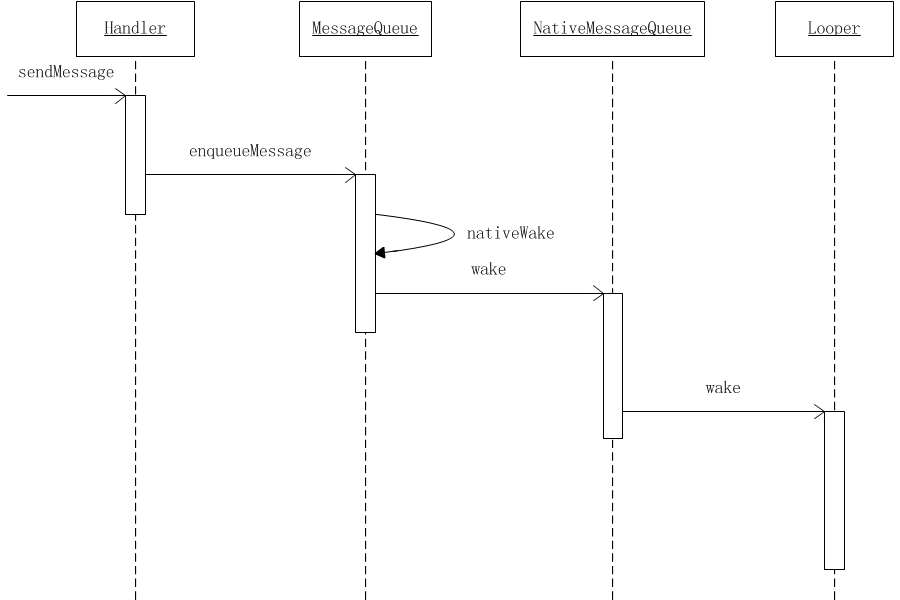
Handler或Post類方法最終都會調用enqueueMessage將消息發送到消息隊列
private boolean enqueueMessage(MessageQueue queue, Message msg, long uptimeMillis) {
msg.target = this;
if (mAsynchronous) {
msg.setAsynchronous(true);
}
return queue.enqueueMessage(msg, uptimeMillis);
}
Message的成員變量target賦值為this,即關聯到handler.然后繼續調用MessageQueue的enqueueMessage方法
boolean enqueueMessage(Message msg, long when) {
if (msg.target == null) {
throw new IllegalArgumentException("Message must have a target.");
}
if (msg.isInUse()) {
throw new IllegalStateException(msg + " This message is already in use.");
}
/// M: Add message protect mechanism @{
if (msg.hasRecycle) {
Log.wtf("MessageQueue", "Warning: message has been recycled. msg=" + msg);
return false;
}
/// Add message protect mechanism @}
synchronized (this) {
if (mQuitting) {
IllegalStateException e = new IllegalStateException(
msg.target + " sending message to a Handler on a dead thread");
Log.w("MessageQueue", e.getMessage(), e);
msg.recycle();
return false;
}
msg.markInUse();
msg.when = when;
Message p = mMessages;
boolean needWake;
if (p == null || when == 0 || when < p.when) {
// New head, wake up the event queue if blocked.
msg.next = p;
mMessages = msg;
needWake = mBlocked;
} else {
// Inserted within the middle of the queue. Usually we don't have to wake
// up the event queue unless there is a barrier at the head of the queue
// and the message is the earliest asynchronous message in the queue.
needWake = mBlocked && p.target == null && msg.isAsynchronous();
Message prev;
for (;;) {
prev = p;
p = p.next;
if (p == null || when < p.when) {
break;
}
if (needWake && p.isAsynchronous()) {
needWake = false;
}
}
msg.next = p; // invariant: p == prev.next
prev.next = msg;
}
// We can assume mPtr != 0 because mQuitting is false.
if (needWake) {
nativeWake(mPtr);
}
}
return true;
}
MessageQueue中的enqueueMessage主要工作是將message插入到隊列,然后根據情況判斷是否應該調用nativeWake喚醒目標線程.當前隊列為空或者插入消息處理時間延時為0或者處理時間小于隊頭處理時間時,消息被插入到頭部,否則按時間遍歷插入到對應位置,并設置needWake標志,needWake是根據mBlocked來判斷的,mBlocked記錄了當前線程是否處于睡眠狀態,如果消息插入隊頭且線程在睡眠中,neeWake為true,調用nativeWake喚醒目標線程.
static void android_os_MessageQueue_nativeWake(JNIEnv* env, jclass clazz, jlong ptr) {
NativeMessageQueue* nativeMessageQueue = reinterpret_cast<NativeMessageQueue*>(ptr);
return nativeMessageQueue->wake();
}
void NativeMessageQueue::wake() {
mLooper->wake();
}
void Looper::wake() {
uint64_t inc = 1;
ssize_t nWrite = TEMP_FAILURE_RETRY(write(mWakeEventFd, &inc, sizeof(uint64_t)));
if (nWrite != sizeof(uint64_t)) {
if (errno != EAGAIN) {
ALOGW("Could not write wake signal, errno=%d", errno);
}
}
}
nativeWake最終會調用到jni層的Looper對象的wake方法中,Looper wake方法的實現非常簡單,即向mWakeEventFd寫入一個uint64_t,這樣目標線程就會因為mWakeEventFd發生的IO事件而喚醒.消息的發送流程就此結束.
線程消息的處理
從前面的分析可以知道,當線程沒有消息需要處理時,會在c++層Looper對象的pollInner中進入睡眠等待,當有新消息喚醒該目標線程時或這延時時間到,執行流程將沿著pollInner調用路徑一直返回,直到java層Looper類的loop.
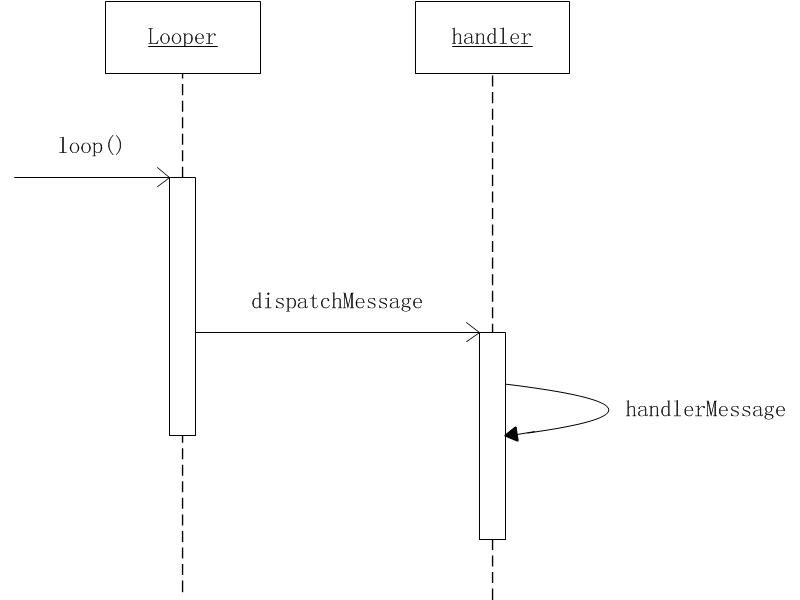
loop中將調用msg.target.dispatchMessage(msg)處理消息,這里的msg.target就是上面enqueueMessage中所賦值的handler,即進入handler的dispatchMessage處理消息
public void dispatchMessage(Message msg) {
if (msg.callback != null) {
handleCallback(msg);
} else {
if (mCallback != null) {
if (mCallback.handleMessage(msg)) {
return;
}
}
handleMessage(msg);
}
}
dispatchMessage進行消息處理,先檢查是否有設置msg.callback,如果有則執行msg.callback處理消息,如果沒有則繼續判斷mCallback的執行,最后才是handleMessage處理.